How to handle static files in Django
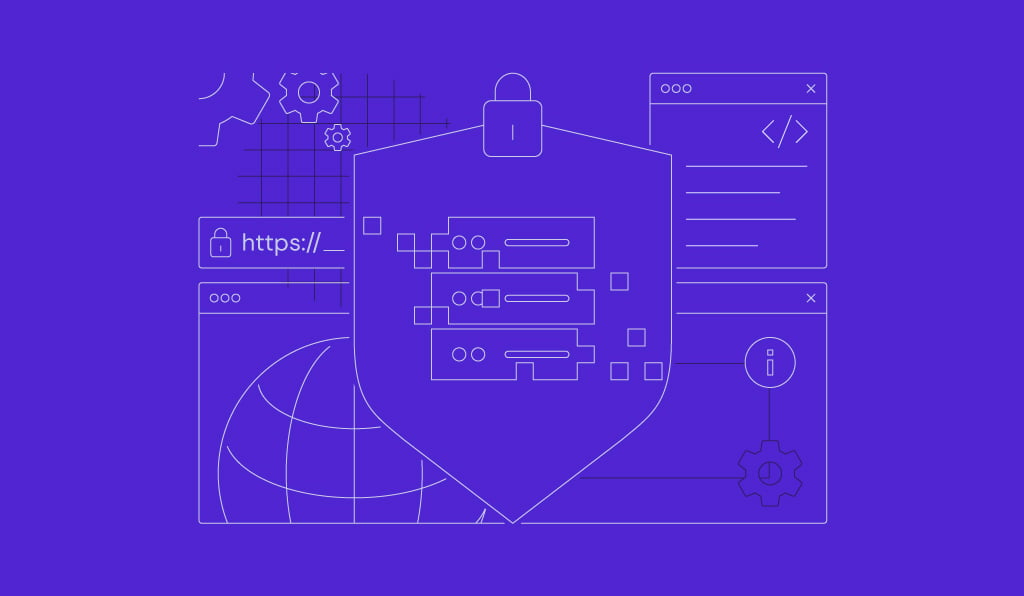
Django provides a simple and efficient way to manage static files, such as CSS, JavaScript, and images, which are an important part of an application or a website.
In this tutorial, we will explain how to handle Django static files to ensure they are properly served and organized during development and deployment.
Setting up static files in Django
Below you’ll find instructions on how you can set up and configure static files.
Configuring static files in settings.py
To manage static files in Django, you need to configure your project to know where to look for and serve them. You can do so by specifying the Static URL and Directory in settings.py.
# settings.py STATIC_URL = '/static/' STATICFILES_DIRS = [ BASE_DIR / "static", # Optional: Add this if you want to store static files in a 'static' folder within your project ]
The STATIC_URL parameter defines the URL prefix Django will use when serving static files. Meanwhile, STATICFILES_DIRS specifies additional directories where Django will look for the files.
App-specific static files
By default, Django will look for static files inside each app’s static directory. For example, if you have an app named myapp, you can create a folder structure like this:
myapp/ │ ├── static/ │ └── myapp/ │ └── style.css └── views.py
Django will automatically recognize and serve these files during development.
Linking static files in templates
To use static files in your Django templates, you must first load Django’s {% static %} template tag. Then, you can reference your static files by their relative paths:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Home Page</title> {% load static %} <link rel="stylesheet" href="{% static 'myapp/style.css' %}"> </head> <body> <h1>Welcome to My Django App</h1> <img src="{% static 'myapp/images/logo.png' %}" alt="Logo"> </body> </html>
Here’s the explanation of the static tags:
- {% load static %}: Loads the static files template tag.
- {% static ‘myapp/style.css’ %}: Resolves the path to the style.css file in the static/myapp directory.
- {% static ‘myapp/images/logo.png’ %}: Resolves the path to an image file.
Collecting static files for deployment
During development, Django serves static files automatically when DEBUG = True. In production, however, you must collect all static files from various locations and store them in a single directory that your web server can find.
Configuring static root directory
To configure the static root directory, Django provides the collectstatic management command.
Before using it, make sure you have included django.contrib.staticfiles in the INSTALLED_APPS setting of settings.py:
INSTALLED_APPS = [ # Other installed apps 'django.contrib.staticfiles', ]
Now, configure the Static Root Directory in settings.py. This is the folder Django will use to collect static files for deployment.
STATIC_ROOT = BASE_DIR / 'staticfiles'
During deployment, Django won’t serve the static files anymore and your web server will take over. If we use the default static directory in the Django app.
Running the collectstatic Command
The collestatic command collects all static files from each app’s static directory and any additional locations specified in STATICFILES_DIRS.Then, it stores them in the STATIC_ROOT directory.
Here’s how to run the collectstatic command:
python manage.py collectstatic
Serving static files during development
After setting up the root static directory and collecting all the files, configure your web server to serve them. Depending on your web server, the configuration might differ.
For example, here’s how the configuration might look for NGINX:
erver { listen 80; server_name yourdomain.com; location /static/ { alias /path/to/your/project/staticfiles/; # The path to STATIC_ROOT } location / { proxy_pass http://127.0.0.1:8000; # Pass requests to Django app } }
In this configuration, NGINX serves static files directly from the /static/ directory while forwarding all other requests to the Django application.
By properly managing static files in Django, you ensure that your application not only looks good with styles and images but also performs efficiently when deployed.
Caching static files
Since static files look the same whenever users access them, caching them is a great strategy for improving your website’s loading speed. However, you must configure it on your web browser since Django can’t serve those files directly.
To enable caching, we must add the cache-control header to tell users’ web browser that they can store the static content for reuse.
For NGINX, you can enable it by adding the following code into your configuration file. Make sure to add this after the alias:
expires 30d; # Cache for 30 days add_header Cache-Control "public, max-age=2592000, immutable";
To further optimize your caching, you can use Django’s ManifestStaticFilesStorage. It hashes your static files, meaning their name will change automatically after you modify their content.
Hashing enables users’ web browsers to store the cache as long as possible until it reaches the maximum age to maintain optimal loading time. Browsers will only download the new files when their name changes.
You can enable ManifestStaticFilesStorage by adding the following code in your settings.py file:
STATICFILES_STORAGE = 'django.contrib.staticfiles.storage.ManifestStaticFilesStorage'
Integrating third-party static libraries
To improve your development efficiency, Django lets you include static files from third-party libraries. For example, you can integrate Bootstrap to add CSS or JQuery for JavaScript.
There are different ways to do so, but manually adding the library files to your Django project is the most common. Moreover, the steps are the same regardless of the library.
For example, here’s how to install Bootstrap in your Django project:
- Download the compiled CSS and JS version of Bootstrap:
wget https://github.com/twbs/bootstrap/releases/download/v4.0.0/bootstrap-4.0.0-dist.zip
- Extract the downloaded archive file using this command:
unzip bootstrap-4.0.0-dist.zip
- Create a static folder inside your Django project’s root directory in the same location as manage.py. Skip this step if you have the folder already.
mkdir myapp/static
- The archive we extracted earlier yielded two folders called css and js. Move them to the static folder using these commands:
mv css myapp/static mv js myapp/static
- Open settings.py using a text or code editor.
- Change the STATIC_URL value to /static/. If you can’t find the parameter, add it manually:
STATIC_URL = '/static/'
- Save the changes.
Now you can load static files from your library by calling them using the {% static %} tag like the following example:
<head> <title>My Django Project</title> <link rel="stylesheet" href="{% static 'css/bootstrap.min.css' %}"> </head>
Conclusion
Static files like CSS, JavaScript, and images are important elements in a web application. In Django, you can manage it efficiently using the built-in features and command utility.
In development, Django static files are located inside your app’s static folder. By editing the settings.py file, you can specify additional folders for storage and set URLs that will serve them. To load static files, use the {% static %} tag in your template.
During deployment, collect the files into the root folder using the collectstatic command, so your web browser can serve them. You can also integrate third-party libraries like JQuery and enable Django’s built-in caching feature to optimize your static content.
How to handle Django static files FAQs
What are static files in Django?
In Django, static files are assets like CSS, JavaScript, and images that are not dynamically generated by the backend but are essential for the front end of a web application. They don’t change with each request, so they’re “static” and are usually served as-is to users.
How to serve static files in Django?
To serve static files in Django, set STATIC_URL and STATICFILES_DIRS in settings.py, which will serve and store the CSS, JavaScript, and images. During development, Django serves these files automatically. In production, run python manage.py collectstatic to collect all static files in STATIC_ROOT for your web server.
Can I use CDN for Django static files?
Yes, you can use a CDN for Django static files by setting STATIC_URL to the CDN URL in settings.py. After running collectstatic to gather files, upload them to the CDN. This approach improves load times and reduces server load by delivering assets through a global network.