What can you do with Python: 5 real-life uses and learning tips
Python is a versatile programming language known for its readability, simplicity, and seamless integration with other languages. Python’s extensive standard library and active community make it a popular choice for developers.
In this article, we’ll focus on several areas Python is most commonly used for and highlight some of its strengths that make it so popular.
Python is also considered a great coding language to start out with if you’re learning how to code. So, to help you out, we’ll recommend some online courses and share practical tips to make your coding journey both effective and enjoyable.
What Python is used for
From building dynamic websites to developing AI models and crawling through massive datasets, Python is incredibly versatile. It can automate repetitive tasks, dig deep into data for insights, and power software across various platforms.
So, let’s take a closer look at how Python is used for web development, automation, data science, machine learning, software testing, and the gaming industry.
Web development
Python is often used to build the server side of web applications. It handles the logic, database interactions, and server configuration.
It has several frameworks that simplify web development by providing pre-built modules and tools, like Django, Bottle, or Flask. They’re ideal for small applications, prototyping, and learning purposes, letting you focus on writing apps rather than the details.
Developers often use Selenium, an open-source set of tools and libraries for browser automation. Selenium has an interface that lets you write test scripts in Python for web scraping dynamic content. Scrapy, an open-source and collaborative framework, is a Python-based web scraping tool used for web indexing and extracting data from websites.
Finally, Python is used to create RESTful APIs, which allow different parts of a web application to communicate with each other.
Here are some commonly used Python frameworks for web development:
- Django: A framework that enables rapid web development through its built-in features, such as the admin panel and authentication system.
- Flask: A flexible framework that gives developers more control over the components they use.
- Pyramid: A web framework that supports both small and large applications, allowing developers to start simple and scale up as needed.
- FastAPI: A high-performance web framework for building APIs with Python.
- Tornado: A framework that handles long-lived connections, making it ideal for real-time applications like WebSockets and chat systems.
If you’re looking to get into web development with Python, you’ll want to learn the basics of HTML and CSS. Knowing a bit about Javascript and front-end libraries like React will come in useful as well.
Once you’re comfortable with your skills, consider VPS hosting to deploy your Python projects using the frameworks listed above. A scalable virtual environment like a VPS offers plenty of opportunities to experiment, iterate, and showcase your work on a professional-looking website.
Data science and machine learning
Python libraries enable efficient data manipulation and statistical modeling in big data processing. This makes Python a suitable choice for large-scale data science projects.
Additionally, Python’s data visualization libraries create detailed and interactive plots, which are essential for data analysis and reporting.

Here are some of the more popular libraries and frameworks used in machine learning:
- Scikit-learn: Predictive analytics and automated decision-making
- TensorFlow with the Keras API: Complex analyses and AI model development
- PyTorch: Flexibility and ease of use in research.
- Pandas and NumPy: Data manipulation and numerical computations
These libraries come with pre-built functions and tools that simplify complex tasks, such as data preprocessing, model training, and evaluation, greatly improving productivity.
If you’re not sure where to get started, you can always check out the Udemy Python for Data Science course from Jose Portilla or the blog of Wes Mckinney, the creator of Pandas. Want to jump straight into machine learning? You’ll want something that will introduce you to Scikit-learn and NumPy, like Andrew Ng’s course on Coursera.
Scripting and automation
Automation with Python involves using scripts to perform repetitive tasks without human intervention. You can automate a wide range of tasks, improving productivity and reducing errors.
Area of automation | How you can boost productivity | Python script examples |
File systems and directory management | Use libraries like OS and Shutil to automate tasks like renaming files, organizing directories, and backing up data. | import osos.rename('old_name.txt', 'new_name.txt') |
Web scraping | Extract data from websites using libraries like BeautifulSoup and Scrapy. | from bs4 import BeautifulSoupimport requestsresponse = requests.get('http://example.com')soup = BeautifulSoup(response.text, 'html.parser') |
Web automation | Automate web interactions such as form submissions and data extraction using Selenium. | from selenium import webdriverdriver = webdriver.Chrome()driver.get('http://example.com') |
System administration | Monitor system resources, manage processes, and automate backups using libraries like psutil. | import psutilprint(psutil.cpu_percent()) |
Email automation | Send emails, manage inboxes, and automate responses using libraries like smtplib and imaplib. | import smtplibserver = smtplib.SMTP('smtp.example.com', 587) |
Data processing | Automate data cleaning, transformation, and analysis using libraries like Pandas and NumPy. | import pandas as pddf = pd.read_csv('data.csv') |
If you want to learn more about using virtual environments to set up an automation sandbox, check out our article on creating a virtual environment using Python.
Software testing
Let’s have a look at typical software testing scenarios and the Python-based tools that support them.
Unit and integration testing
Different software testing processes verify whether individual units of code work as expected and how they interact with other software system modules.
Python’s built-in library of choice for writing and running tests is unittest. It supports test automation and makes the tests independent from the framework they report to. Pytest is a more flexible testing framework that supports more advanced testing and plugins.
Pytest is often used for integration testing thanks to its ability to handle complex test scenarios and its extensive plugin ecosystem. Nose2 is an extension of unittest that supports plugins and is used for larger-scale integration tests.
Behavior-Driven Development (BDD)
Behavior-Driven Development (BDD) is an Agile software development approach that aims to create a common understanding of software behavior. Python tests can be created and executed to verify that the software behaves as expected.
Python offers frameworks designed to support BDD, like Behave or Lettuce. With these frameworks, DevOps engineers can write tests in a natural language style, making it easier for non-developers to understand.
Continuous Integration (CI)
CI is the primary method for collaborating and integrating code in software development. It allows developers to frequently merge code changes into a central repository, where builds run and tests are performed.
Jenkins is a platform often used with Python scripts to automate the testing process in CI pipelines. Tox is a tool used for automating testing in multiple environments, ensuring that code works across different Python versions and dependencies.
Web testing
Web testing evaluates a web application’s functionality, usability, security, compatibility, and performance.
Webmasters use Selenium to automate web browsers. Python, combined with Selenium, allows them to write scripts that can navigate web pages, fill out forms, click buttons, and verify results. Requests is a library for making HTTP requests and is useful for testing APIs.
Performance testing
Performance testing checks how a system performs regarding responsiveness and stability when tested under varying workload conditions.
Locust is an open-source load-testing tool written in Python code that allows you to define user behavior and simulate millions of users.
JMeter is a good example of how well Python integrates with other languages since this Apache application can be extended with Python scripts for more complex performance testing scenarios.
Game development
Python is an excellent choice for developing games, as it can quickly produce prototypes and efficiently iterate on early game ideas. For example, Renpy, a popular game engine for creating interactive visual novels, uses Python at its core.
Game developers often use Python for auxiliary systems, like level editors, asset managers, and testing frameworks. For instance, Battlefield 2 used Python for its multiplayer infrastructure and network communication.
Modding is often made possible through frameworks built on Python. However, other languages or engines are more likely to be used for resource-intensive or mobile games.
Python offers a suite of libraries that enable the creation of a wide variety of games:
- Pygame: A popular library for creating 2D games. It provides functionality for handling graphics, sound, and user input.
- Pyglet: Another library for 2D games, known for its performance and ease of use.
- Panda3D: A game engine that supports 3D rendering and game development.

Integrating intelligent NPCs and procedural content into video games is made easier because machine learning, AI development, and other technologies are typically done in Python.
Game developers who use Python to create games can benefit from automating tasks or creating game logic in engines like Unity and Unreal. Keep in mind that you will need to install a plugin for Unreal and a separate third-party package for Unity.
If you’re not looking to create your game engine from scratch or don’t want to install compatibility plugins, check out Python-compatible engines like Godot. It’s completely free and open-source, with a dedicated community that provides a constant stream of updates and new features.
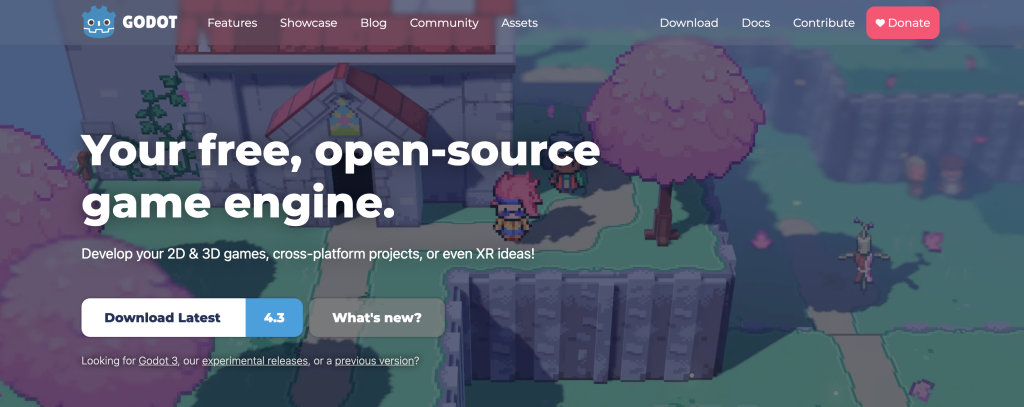
Godot’s native code language is GDScript, which uses Python syntax. If you’re familiar with Python, you will only need to make a few changes when writing code. The benefits of using an existing game engine include intuitive GUIs, user-made plugins, hassle-free exporting and platform support, and much time saved by not having to write your own infrastructure.
Python’s limitations in game development
Python is generally slower than languages like C++ or C#, which can be a drawback for performance-intensive games. While libraries like Panda3D exist, Python is not as strong in 3D game development as other languages or engines.
A significant hurdle for Python’s adoption in game creation is its automatic memory management, which may lead to inefficiencies. For these reasons, most high-budget AAA games are not developed with Python, making the language less common in the mainstream game development industry.
Tips for learning Python
To start learning Python, consider enrolling in an online course – there are plenty of free and paid options available.
We’ve selected a few examples that we believe are great choices to start with:
- HarvardX: CS50’s Introduction to Programming with Python is a comprehensive and free online course that brings you closer to general-purpose programming using Python.
- Python Mega Course is a paid course on the Udemy learning platform that teaches you Python skills through building real programs.
- Codecademy offers plenty of Python courses, along with an interactive learning style that includes quizzes and DIY project challenges

Once you choose the right course, follow these ground rules to make the lessons stick:
- Practice consistently, even daily, to build familiarity.
- Focus on core concepts like variables and analyze data types, loops, and functions.
- Write down code snippets and explanations by hand.
- Use interactive tools like Jupyter Notebook and platforms like Codecademy.
- Apply your knowledge practically, and create small projects of your own, like a calculator or a web scraper.
- Join Python communities and engage with forums, social media groups, and meetups.
- Learn to use Python’s debugging tools and understand common errors.
- Explain concepts to others to reinforce your understanding.
- Familiarize yourself with popular libraries like Pandas, NumPy, Flask, and Django.
- Keep up with the latest Python developments and updates.
If you feel like you’d learn faster in a community setting, consider joining a group course or even the community-made Python Discord. Events like hackathons and code jams can be a great way to cooperate with others and improve your own skills.
And remember, if you get stuck – take a break, work on a side project or another skill. Avoiding burnout is a crucial step when learning anything, and a coding language is no exception.
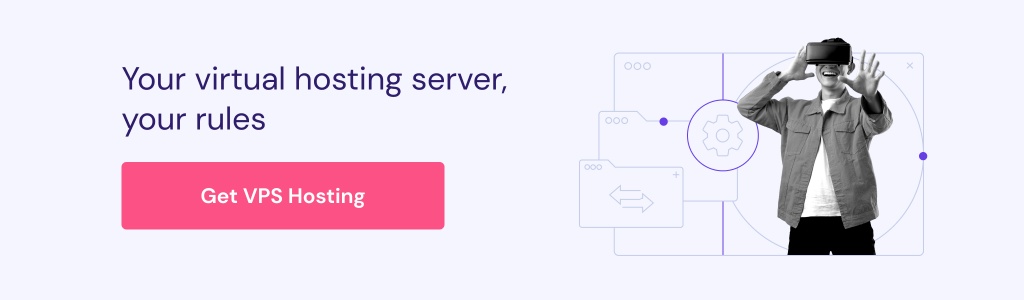
Conclusion
Python is a well-established language with many useful libraries and a large community of skilled developers. It’s also easy to learn because its syntax is straightforward and readable. This makes Python a top pick for both beginners and experts, especially for complex projects.
Being one of the most popular programming languages, it is used in some of the most influential fields, from web development through automation and testing to data science or game development.
If you want to learn Python, make sure to spend some time choosing the right course. Coding takes time and effort, so make sure you practice the core concepts that you’re learning. Stay engaged with the community to be on top of best practices and useful tools for Python coding.
If you have any more questions, have a look at the FAQ section or leave a comment below.
What can you do with Python FAQ
Is Python difficult to learn?
Python is great for beginners – its simple syntax and minimal code make Python accessible and user-friendly. Plus, Python has a large and supportive community, with many educational resources available – courses, books, and interactive coding platforms – all of which support a variety of learning styles.
What jobs require Python knowledge?
Python is a highly versatile language used in a multitude of fields, like web development, data science, machine learning, scientific research, and DevOps.
From building websites to analyzing data and developing AI, Python skills are in high demand across many industries.
Is Python better than C++?
Both languages have their strengths and are suited to different types of tasks.
Python can be better for web development, data analysis, machine learning, or automation scripts. C++ is great for game development, system or software development, real-time simulations, and high-performance applications.
Python’s strengths lean towards faster development, enabling quick prototyping through lean code. Python also works on various operating systems with minimal changes. Have a look at our tutorial on running Python scripts in Linux.